Table 3287. Cheatsheet of numpy.
Code |
Output |
Explaination |
a = np.array([1, 2, 3, 4, 5])
print(a) |
[1 2 3 4 5] |
Array (Code) |
a = np.array([1, 2, 3, 4, 5])
print(type(a)) |
<class 'numpy.ndarray'> |
(Code) |
a = np.array([1, 2, 3, 4, 5])
a[0] = 120
print(a) |
[120 2 3 4 5] |
Change the first element or more (Code) |
a = np.array([1, 2, 3, 4, 5]) a[2:4] = 300, 500
print(a) |
[120 2 300 500 5] |
Change multiple elements (Code) |
a = np.array([1, 2, 3, 4, 5])
d = a[1:3]
print(d) |
[ 2 300] |
Select elements and assign to a new array (Code) |
print(np.zeros((3,4))
) |
[[0. 0. 0. 0.]
[0. 0. 0. 0.]
[0. 0. 0. 0.]] |
Create an array of zeros |
print(np.ones((2,3,4),dtype=np.int16)) |
[[[1 1 1 1]
[1 1 1 1]
[1 1 1 1]]
[[1 1 1 1]
[1 1 1 1]
[1 1 1 1]]] |
Create an array of ones |
x = np.arange(1, 12)
print(x) |
[ 1 2 3 4 5 6 7 8 9 10 11] |
|
d = np.arange(10,25,5)
print(d) |
[10 15 20] |
Create an array of evenly spaced values (step value ) |
x = np.arange(1, 13)
print(x)
x = x.reshape(3, 4)
print(x) |
[ 1 2 3 4 5 6 7 8 9 10 11 12]
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]] |
|
x = np.arange(1, 13)
print(x)
x = x.reshape(-1, 4)
print(x) |
[ 1 2 3 4 5 6 7 8 9 10 11 12]
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]] |
|
x = np.arange(1, 13)
print(x)
x = x.reshape(-1, 4)
print(x)
x = x.ravel()
print(x) |
[ 1 2 3 4 5 6 7 8 9 10 11 12]
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]]
[ 1 2 3 4 5 6 7 8 9 10 11 12] |
Back to 1D |
x = np.arange(1, 13)
print(x)
y = np.arange(13, 25)
print(y)
z = np.stack((x, y))
print(z) |
[ 1 2 3 4 5 6 7 8 9 10 11 12]
[13 14 15 16 17 18 19 20 21 22 23 24]
[[ 1 2 3 4 5 6 7 8 9 10 11 12]
[13 14 15 16 17 18 19 20 21 22 23 24]] |
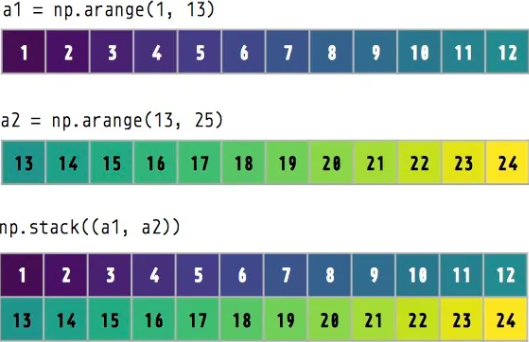 |
# 3D array from 2D array
x = np.arange(1, 13).reshape(3, 4)
print(x)
print()
y = np.arange(13, 25).reshape(3, -1)
print(y)
print()
# Stack along axis 2
z = np.stack((x,y), axis = 2)
print(z)
|
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]]
[[13 14 15 16]
[17 18 19 20]
[21 22 23 24]]
[[[ 1 13]
[ 2 14]
[ 3 15]
[ 4 16]]
[[ 5 17]
[ 6 18]
[ 7 19]
[ 8 20]]
[[ 9 21]
[10 22]
[11 23]
[12 24]]] |
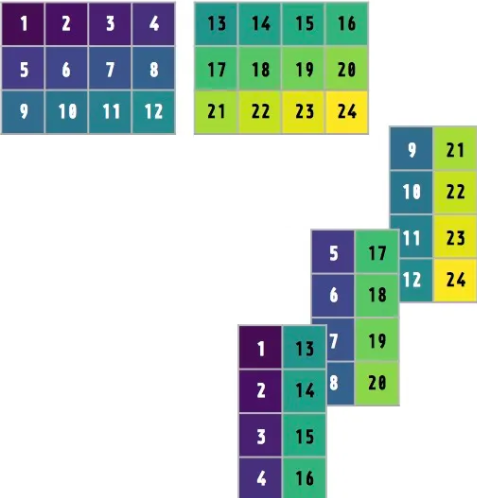 |
x = np.arange(1, 13)
print(x)
y = np.arange(13, 25)
print(y)
z = np.hstack((x, y))
print(z) |
[ 1 2 3 4 5 6 7 8 9 10 11 12]
[13 14 15 16 17 18 19 20 21 22 23 24]
[ 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24] |
|
x = np.arange(1, 13)
print(x)
y = np.arange(13, 25)
print(y)
z = np.stack((x, y), axis =1)
print(z) |
[ 1 2 3 4 5 6 7 8 9 10 11 12]
[13 14 15 16 17 18 19 20 21 22 23 24]
[[ 1 13]
[ 2 14]
[ 3 15]
[ 4 16]
[ 5 17]
[ 6 18]
[ 7 19]
[ 8 20]
[ 9 21]
[10 22]
[11 23]
[12 24]] |
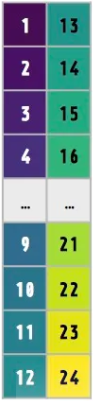 |
print(np.linspace(0,2,9)
) |
[0. 0.25 0.5 0.75 1. 1.25 1.5 1.75 2. ] |
Create an array of evenly spaced values (number of samples ) |
e = np.full((2,2),7)
print(e) |
[[7 7]
[7 7]] |
Create a constant array |
f = np.eye(2)
print(f) |
[[1. 0.]
[0. 1.]] |
Create a 2 X2 identity matrix |
print(np.random.random((2,2))) |
[[0.71539128 0.12045412]
[0.680891 0.59698397]] |
Create an array with random values |
print(np.empty((3,2)) |
[[-1.44037874e-311 1.03565422e+126]
[ 1.44037234e-311 1.44037234e-311]
[ 2.96439388e-323 7.41098469e-323]] |
Create an empty array |
np.save("my_array", a) |
|
Saving & loading on disk |
np.savez( "array.npz", a, b) |
|
Saving & loading on disk |
np.load("my_array.npy") |
|
Saving & loading on disk |
np.loadtxt("myfile.txt") |
|
Saving & loading text tiles |
np.genfromtxt("my_file.csv", delimiter=",") |
|
Saving & loading text tiles |
np.savetxt("myarray.txt", a, delimiter=" ") |
|
Saving & loading text tiles |
e = np.full((2,2),7)
print(e)
print(e.shape) |
[[7 7]
[7 7]]
(2, 2) |
Array dimensions |
e = np.full((2,2),7)
print(e)
print(len(e)) |
[[7 7]
[7 7]]
2 |
Length of array |
e = np.full((2,2),7)
print(e)
print(e.ndim) |
[[7 7]
[7 7]]
2 |
Number of array dimensions |
e = np.full((2,2),7)
print(e)
print(e.size) |
[[7 7]
[7 7]]
4 |
Number of array elements |
e = np.full((2,2),7)
print(e)
print(e.dtype) |
[[7 7]
[7 7]]
int32 |
Data type of array elements |
e = np.full((2,2),7)
print(e)
print(e.dtype.name) |
[[7 7]
[7 7]]
int32 |
Name of data type |
e = np.full((2,2),7)
print(e)
print(e.astype(int)) |
[[7 7]
[7 7]]
[[7 7]
[7 7]] |
Convert an array to a different type |
print(np.info(np.ndarray.dtype) ) |
type(x.dtype)
<type 'numpy.dtype'>
None |
Asking for help |
np.int64 |
|
Signed 64-bit integer types |
np.float32 |
|
Standard double-precision floating point |
np.complex |
|
Complex numbers represented by 128 floats |
np.bool |
|
Boolean type storing TRUE and FALSE values |
np.object |
|
Python object type |
np.string_ |
|
Fixed-length string type |
np.object |
|
Python object type |
np.string_ |
|
Fixed-length string type |
np.object |
|
Python object type |
np.string_ |
|
Fixed-length string type |
np.unicode_ |
|
Fixed-length unicode type |
g = a - b |
|
Subtraction |
np.subtract(a,b) |
|
Subtraction |
a + b |
|
Addition |
np.add(b,a) |
|
Addition |
np.divide(a,b) |
|
Division |
a * b |
|
Multiplication |
np.multiply(a,b) |
|
Multiplication |
np.exp(b) |
|
Exponentiation |
np.sqrt(b) |
|
Square root |
np.sin(a) |
|
Print sines of an array |
np.cos(b) |
|
Elementwise cosine |
np.log(a) |
|
Elementwise natural logarithm |
e.dot(f) |
|
Dot product |
a == b |
|
Elementwise comparison |
e = np.full((2,2),7)
print(e)
print(e < 2) |
[[7 7]
[7 7]]
[[False False]
[False False]] |
Elementwise comparison |
np.array_e qual(a, b) |
|
Arraywise comparison |
a.sum() |
|
Array-wise sum |
a.min() |
|
Array-wise minimum value |
e = np.full((2,2),7)
print(e)
print(e.max(axis = 0)) |
[[7 7]
[7 7]]
[7 7] |
Maximum value of an array row |
b.cumsum(axis=1) |
|
Cumulative sum of the elements |
u = np.array([1,2, 2, 3])
mean_u = u.mean()
print(mean_u) |
2.0 |
Mean |
np.median(b) |
|
Median |
e = np.full((2,2),7)
print(e)
print(np.corrcoef(e)) |
[[7 7]
[7 7]]
[[nan nan]
[nan nan]] |
Correlation coefficient |
np.std(b) |
|
Standard deviation |
x = np.array([0, np.pi])
print(x) |
[0. 3.14159265] |
Π, π |
sin(x) |
|
|
h = a.view() |
|
Create a vie w of the array with the same data |
np.copy(a) |
|
Create a copy of the array |
h = a.copy() |
|
Create a deep copy of the array |
e = np.linspace(0,2,9)
print(e)
print(e.sort()) |
[0. 0.25 0.5 0.75 1. 1.25 1.5 1.75 2. ]
None |
Sort an array |
e = np.linspace(0,2,9)
print(e)
print(e.sort(axis=0)) |
[0. 0.25 0.5 0.75 1. 1.25 1.5 1.75 2. ]
None |
Sort the elements of an array 's axis |
u = np.array([1,0])
v = np.array([0,1])
z = u+v
print(z) |
[1 1] |
Addition |
u = np.array([1,0])
v = np.array([0,1])
z = []
for n, m in zip(u,v):
z.append(n+m)
print(z) |
[1 1] |
Addition: much faster method of addition |
u = np.array([1,2])
v = np.array([3,1])
z = np.dot(u, v)
print(z) |
5 |
Dot product |
u = np.array([1,2, 2, 3])
z = u+1
print(z) |
[2 3 3 4] |
Add a constant to a numpy array: It adds the constant to each element |